Power Automate: Remove Special Characters From A String
When working with string in PowerAutomate we often have to remove special characters from the string. Some common use cases include field cleanup, file creation, etc.
Let's look at building a reusable flow that can replace the special characters from a string easily.
The flow has the following steps:
- Define input parameters for the flow
- specialCharactersToRemove: Define a string variable that stores the special characters you would like to replace
- targetTextToClean: The string that has the special characters that need to be cleaned
- Data transformations to remove the special characters specified in the input parameters specialCharactersToRemove from targetTextToClean.
- Return the cleaned text to the calling flow.
Define Input Parameters
First, we create an instant cloud flow that we can reuse in other flows.
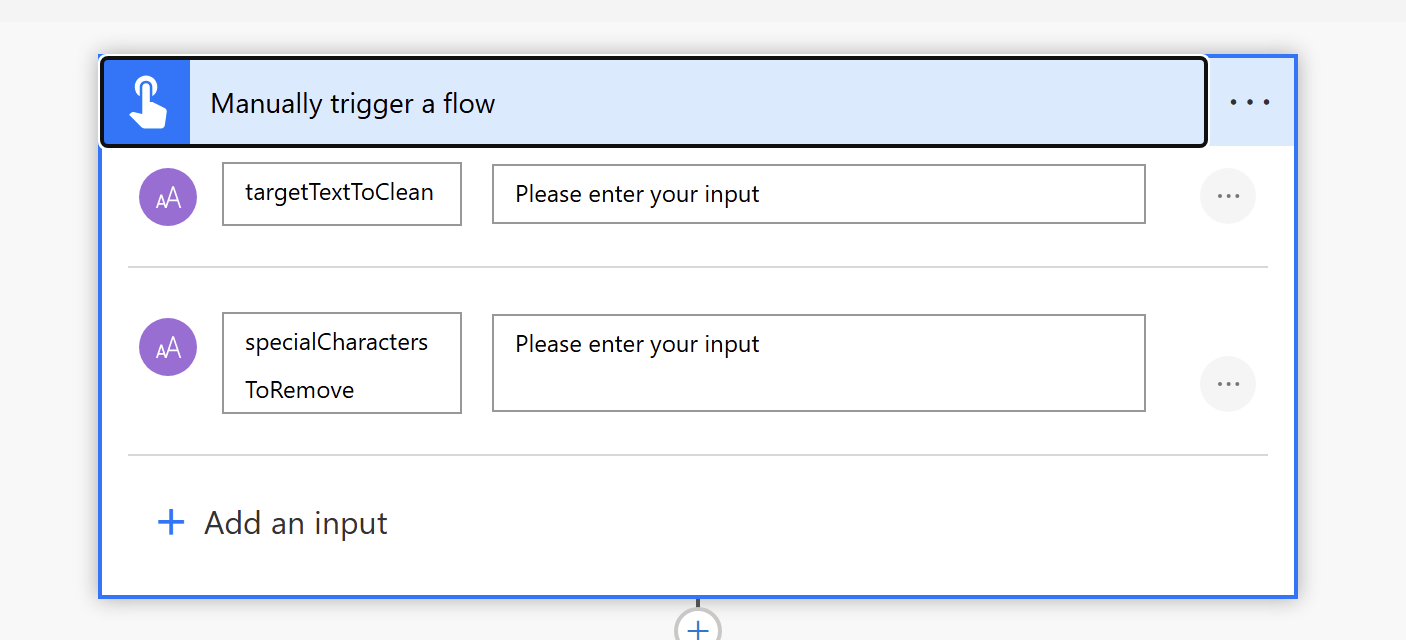
Data Transformations
Now let's look at the data transformations with a simple example
targetTextToClean=a!r
specialCharactersToRemove=!
cleanedText=ar
To achieve the above we can take many approaches. Here is the approach we will be using.
We can achieve this in the flow using the Select data action and a few formulas. First, let's see how the inputs from the flow are represented.
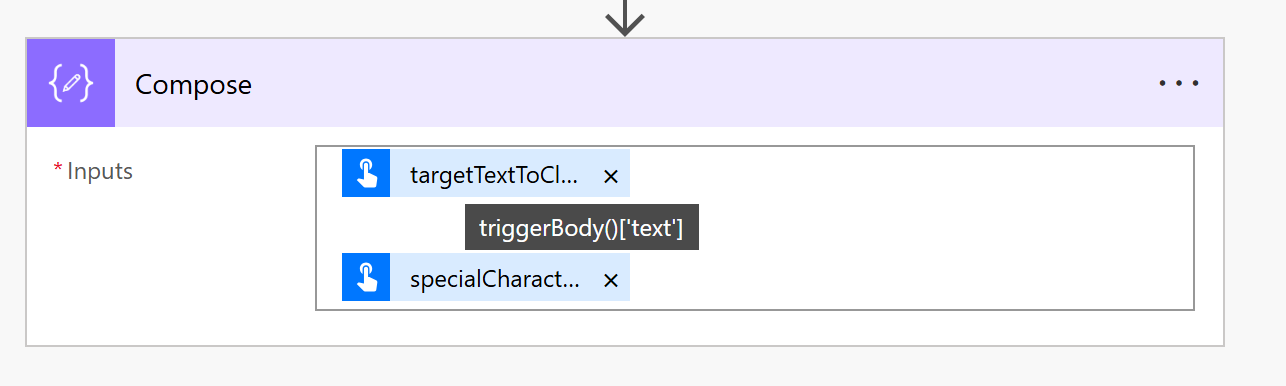
So, anytime we refer to the below formulas, note that we are referring to the targetTextToClean
and specialCharactersToRemove
targetTextToClean = triggerBody()['text']
specialCharactersToRemove = triggerBody()['text_1']
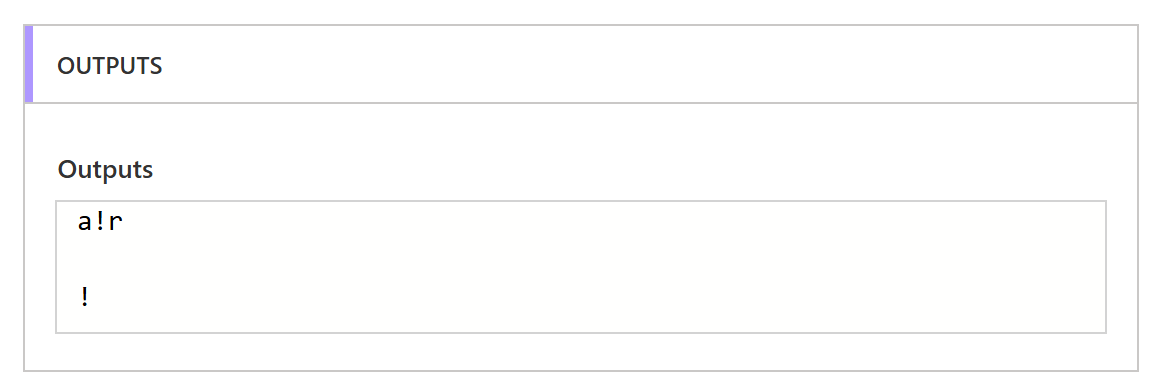
length
: To get the length of the string targetTextToClean
. In the above example, length would return 3.
range
: we create an array with the number of characters in the text. In the above example, a!r
has three characters, so we set the range from (0,3). We set the start index at 0 as the arrays usually start at 0. This would produce an array as [0,1,2]
for the 3 characters we need to account for.
select
: Using the integer array we created, we will use the select action to loop through each integer in the array and find the character at that integer index in the targetTextToClean
.
For the From, we use the below formula
range(0,length(triggerBody()['text']))

item
: Gets the current integer index from the integer array when looping through using select.
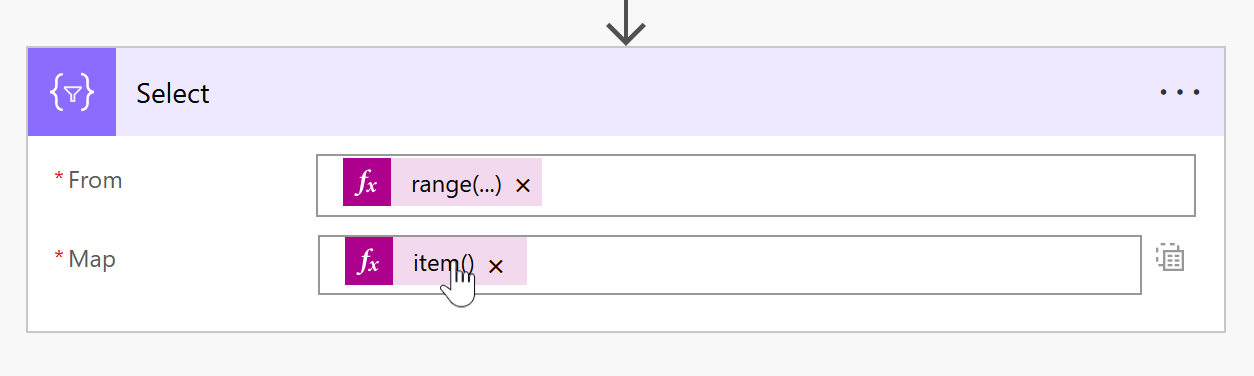
//This will yield the below values for each item in the array
[0,1,2]
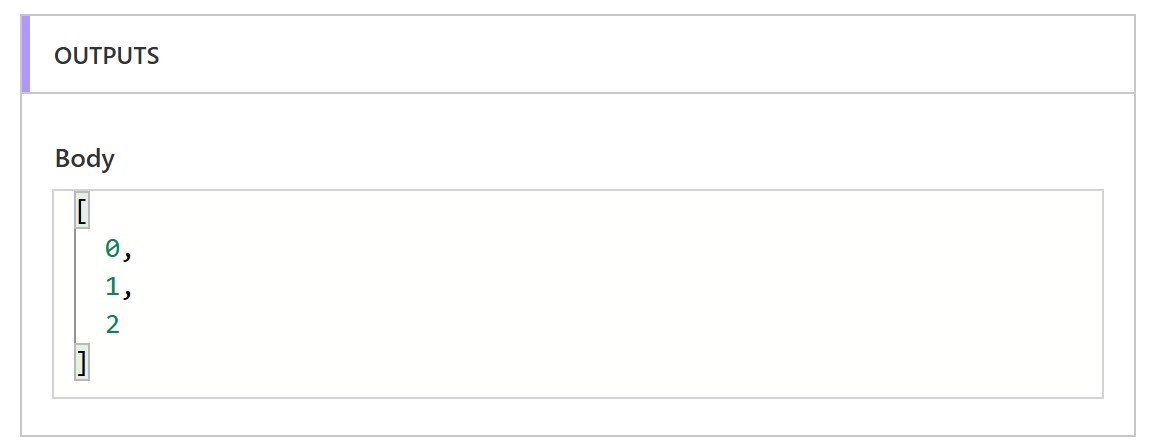
substring
: To find the character at the current index position from the targetTextToClean
. Setting the value of 1 for the length parameter of substring formula ensures only one character is returned.
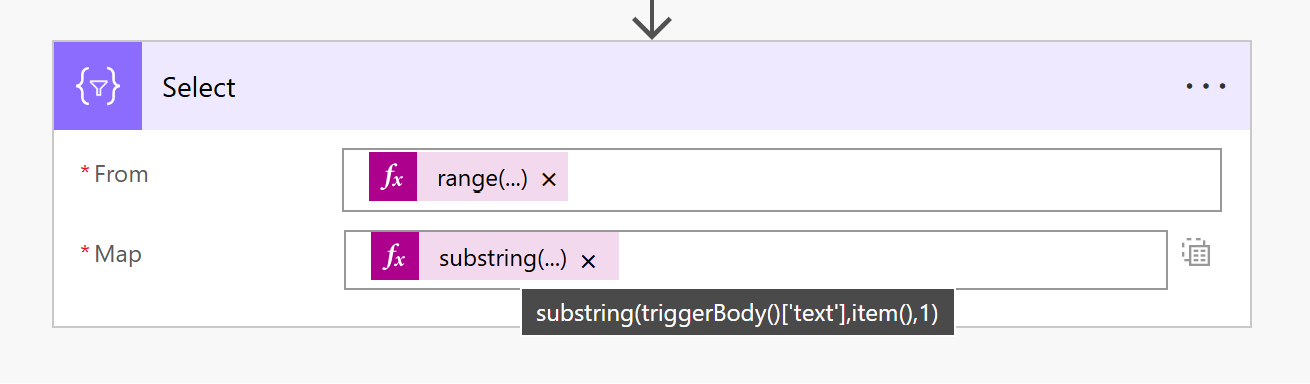
substring(triggerBody()['text'],item(),1)
//In the above example, this will yield the below array if we leave it as is
[a,!,r]
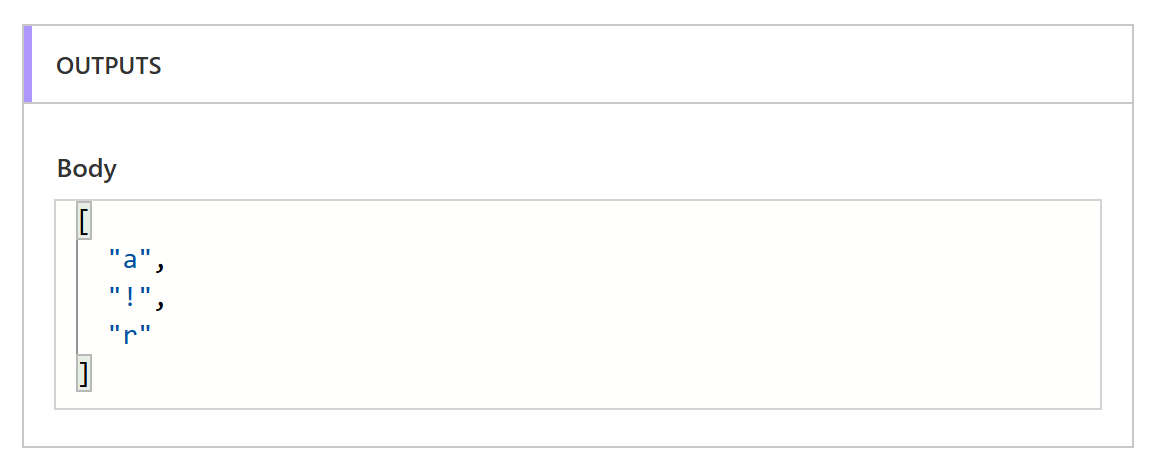
contains
: Check if the specialCharactersToRemove
string contains the character in the current index of the array
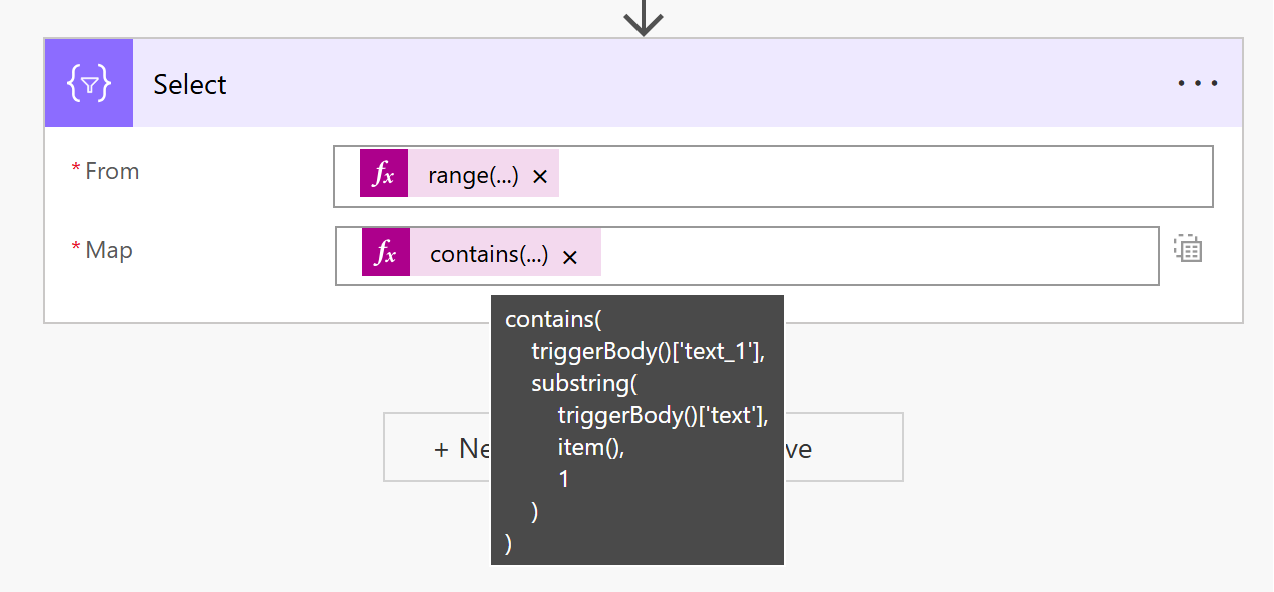
//Check if the specialCharactersToRemove contains the current character
contains(
triggerBody()['text_1'],
substring(
triggerBody()['text'],
item(),
1
)
)
//Output will return as follows
//false - when substring formula returns a
//true - when substring formula returns !
//false - when substring formula returns r
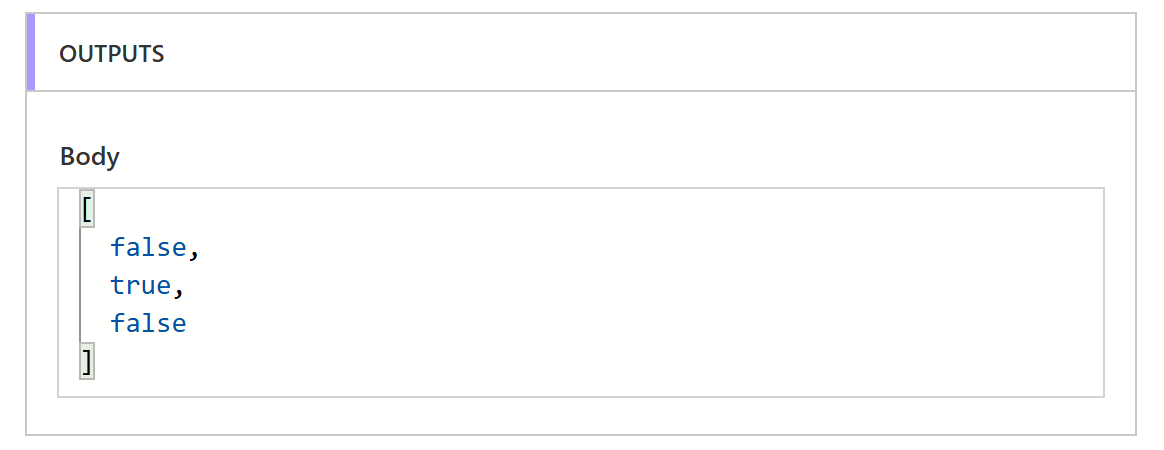
if
: If the current character is character, replace it with empty text. In all other cases, we take the character.
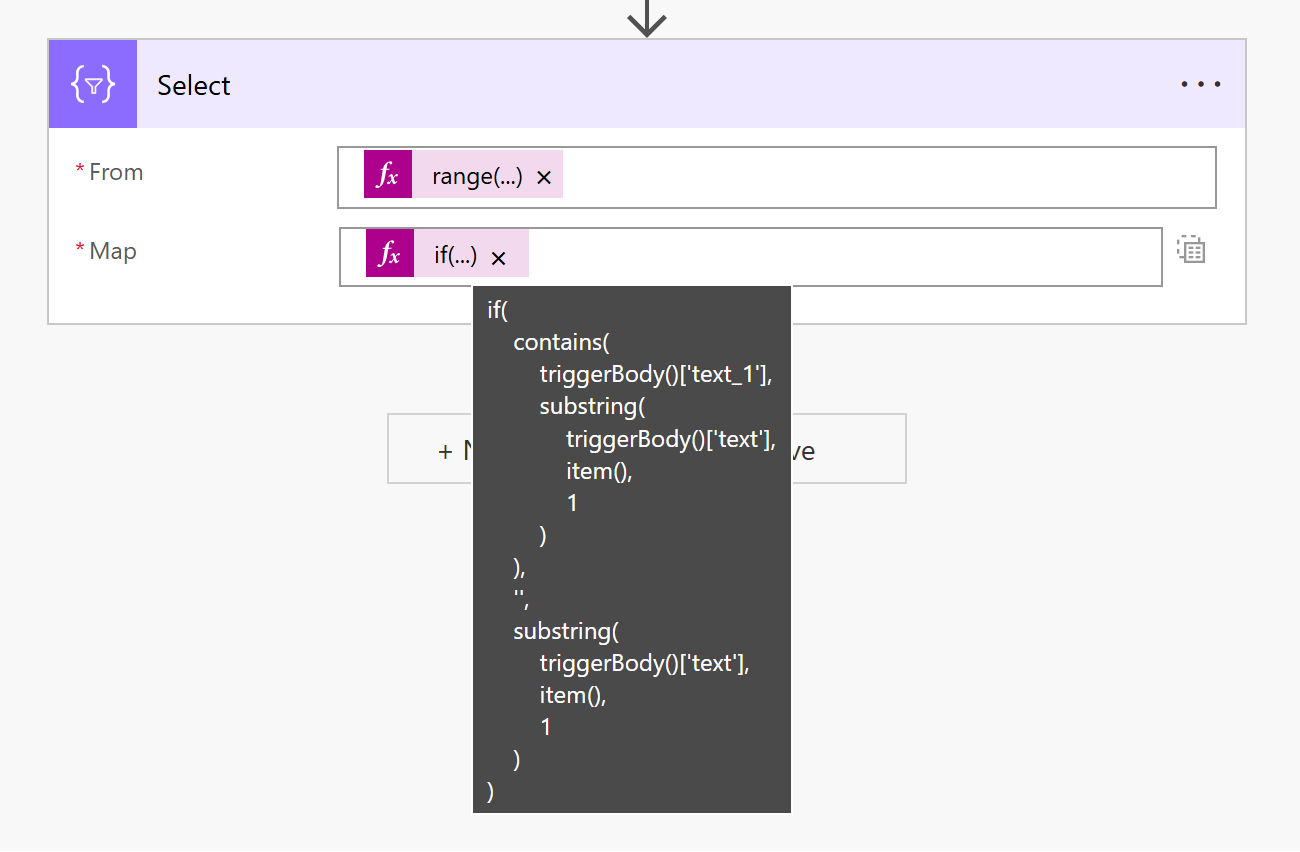
//Returns empty character when it's a special character that needs to be replaced.
if(
contains(
triggerBody()['text_1'],
substring(
triggerBody()['text'],
item(),
1
)
),
'',
substring(
triggerBody()['text'],
item(),
1
)
)
//Outputs the array [a,,r]
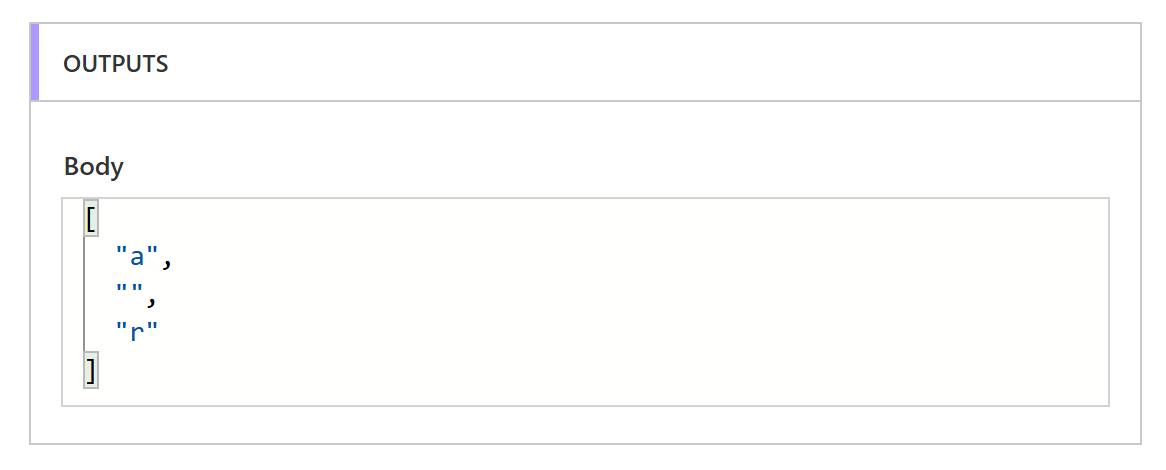
join
: Finally, we use the join formula to combine all the characters from the array into a single string.
I have cleaned the name of the compose operation for this last step as shown below.
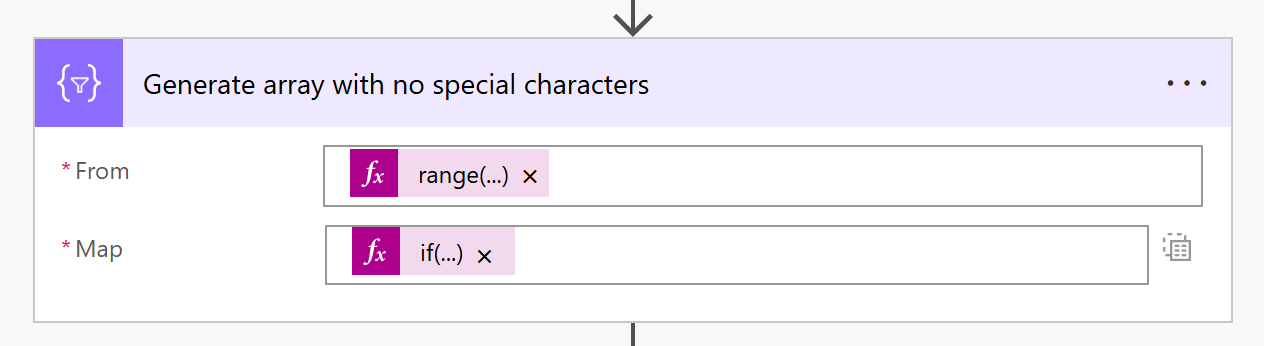
//Converts the array into the string
join(
body('Generate_array_with_no_special_characters'),
''
)
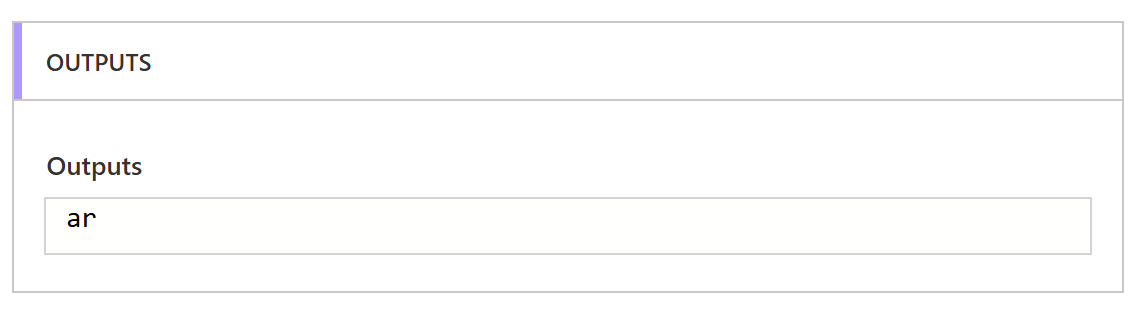
Returning Response
Now our flow is almost complete. The only remaining step is to return the cleaned text as a response to the calling flow. We can return it as JSON so it's easier to access the property in the parent flow. In addition, it's simpler to just add more properties if needed into the JSON in the future.
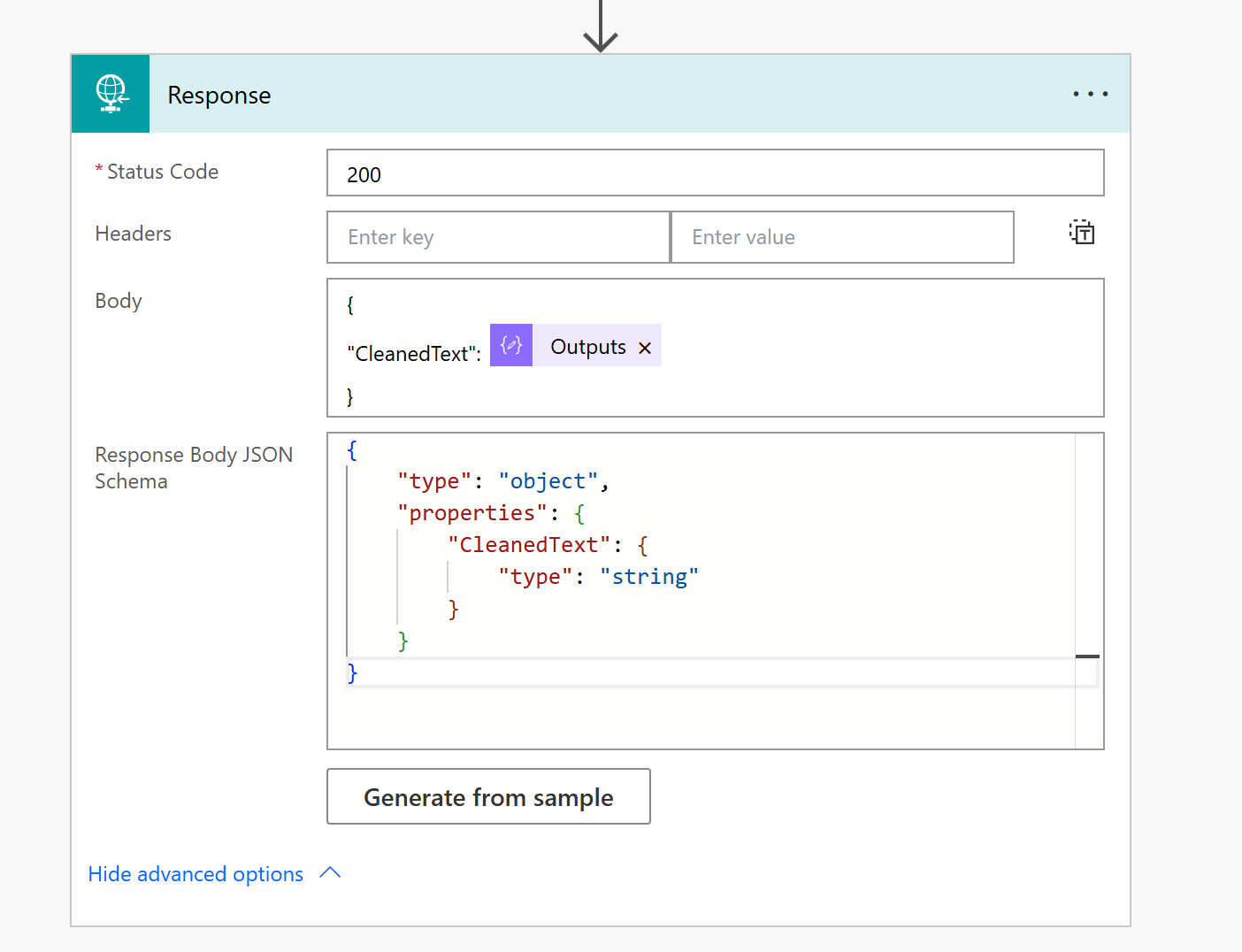
//JSON Schema
{
"type": "object",
"properties": {
"CleanedText": {
"type": "string"
}
}
}
Here is how the flow looks now with all the steps.
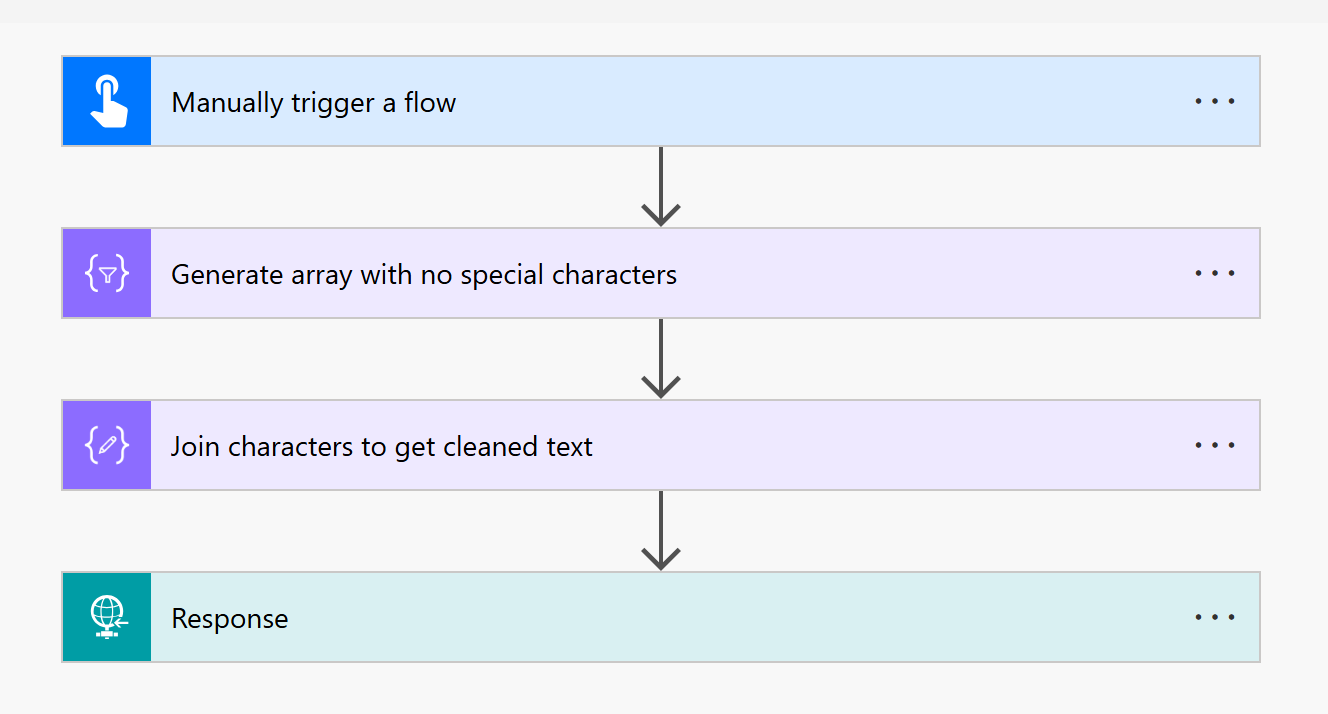
Testing the Flow
Let's test the flow now with complex text and additional characters that need to be removed. As we can see the flow returns the CleanedText as expected.
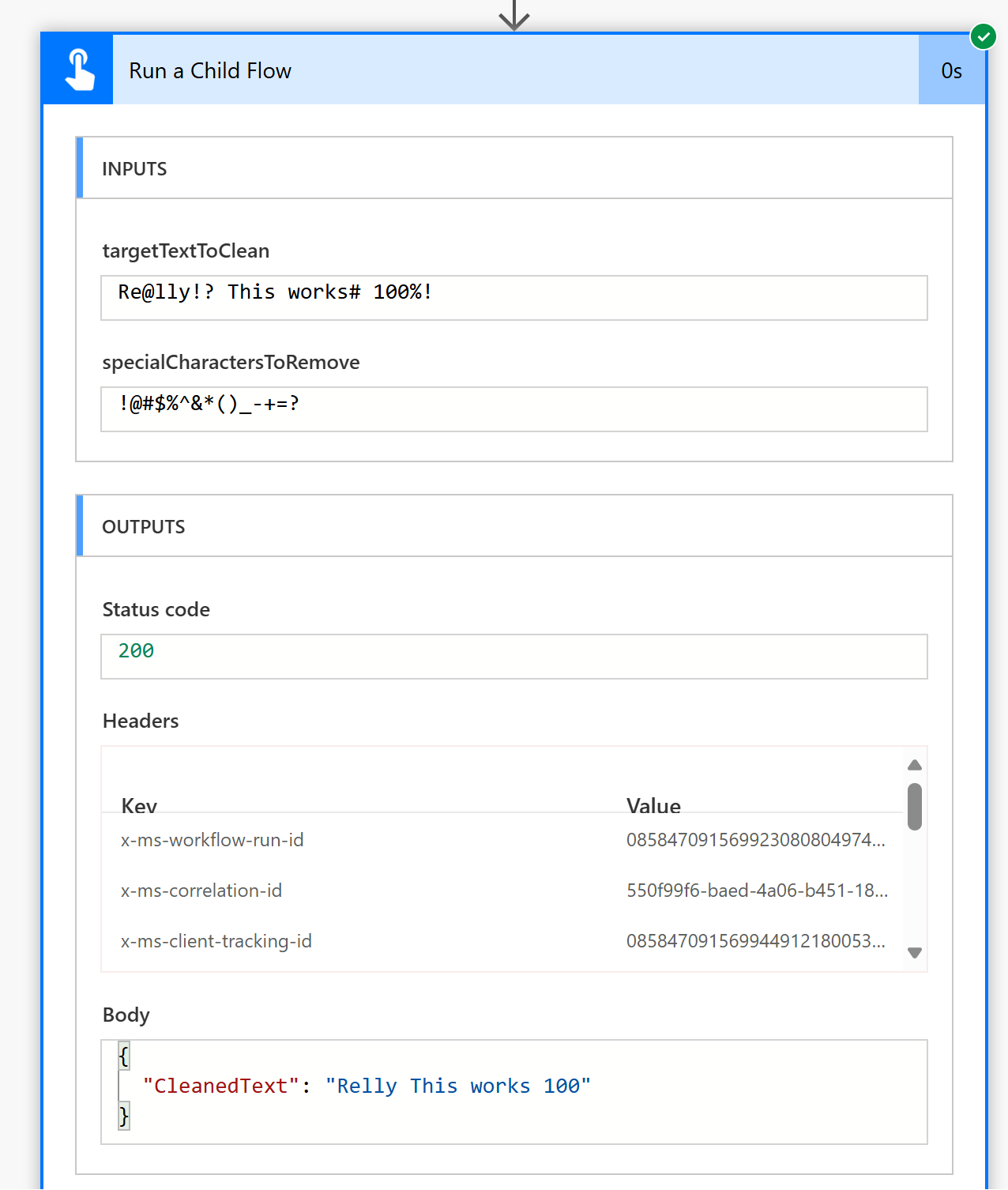